Core Animation for Kiosk Apps
A local non-profit youth football league had a fundraiser this weekend, called Marathon Flag Football. The event set a new world record for the longest continually-played flag football game — over 24 hours. I wrote the game clock, and it turns out Core Animation is fantastic for this.The display was a 720p plasma HDTV, so I just wrote the app to run fullscreen in 1280x720 on the secondary monitor. The great thing about writing a standalone app like this is that you can just pretty much go crazy — no menus, help system, etc.
Because this is a plasma screen, I set up a screensaver to switch on once in a while to prevent burn in. But why just swap images out when you can do it the fun way?
Basically, the app creates about 40 "mosaic" layers on launch, but they're initially offscreen. When the screensaver timer fires, I move them to their place in the grid by adjusting the position attribute, but also make them spin a bit in 3D space using a CATransform3D. Those two animations combined give the impression of paper rising up to the top of the screen, then floating back down.
First, to make any of this work at all, you have to add a perspective transform to the parent layer that contains the individual mosaic tiles. This is what "turns on" 3D space for them. It's important to set this as the sublayerTransform property on the parent — not just transform — because you want the child layers to be rotated, not the parent itself.
// activate depth
CGFloat zDistance = 2000.0;
CATransform3D sublayerTransform = CATransform3DIdentity;
sublayerTransform.m34 = 1.0 / -zDistance;
mosiacConainerLayer.sublayerTransform = sublayerTransform;
The zDistance changes how much the child layer moves in or out of the Z plane (into the monitor) when you change the zPosition property of a layer. We don't do that here, though, so the actual value of the zDistance variable above doesn't matter much in this case. It just has to be something reasonable.
After the sublayerTransform is applied to the parent, we set a new value for the transform property (not sublayerTransform) of each child layer whenever we want to rotate them. This triggers an implicit animation — meaning the animation happens automatically when you change the transform property:
CGFloat rotation = 128.0;
CGFloat x = 54.0;
CGFloat y = 48.0;
CGFloat z = 0.0;
CATransform3D transform;
transform = CATransform3DMakeRotation((rotation * M_PI/180), x, y, z);
childLayer.transform = transform;
To return them to their original 3D orientation, I just set the transform to the CATransform3DIdentity constant. And to get them back to their location in 2D space, I use CALayer's generic key-value coding support to embed the original coordinates in the layer itself without subclassing:
[mosiacTileLayer setValue:[NSNumber numberWithFloat:xPosition]
forKey:@"originalY"];
...
// xPosition is already defined elsewhere
oneLayer.transform = CATransform3DIdentity;
CGFloat xPosition;
CGFloat yPosition = [[oneLayer valueForKey:@"originalY"] floatValue];
oneLayer.position = CGPointMake (yPosition, xPosition);
And yes, even a single-use kiosk app needs an icon.
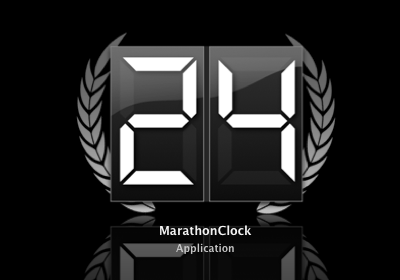
By the way, this event was what this site design was created for.

Core Animation for Kiosk Apps
Posted Sep 1, 2008 — 14 comments below
Posted Sep 1, 2008 — 14 comments below
twobyte — Sep 01, 08 6320
petekaz — Sep 01, 08 6321
Do you have any recommendations in terms of improving one's design skills? I've recently started to get started in Mac development, and although I have been programming for years on the server-side, it seems to succeed on the Mac front, one must build apps that are beautiful. Sadly, I feel my creative side leaves much to be desired. What is the aspiring Mac developer to do?
Scott Stevenson — Sep 01, 08 6323
I'm not really an icon expert at all, but I used Photoshop and Illustrator in this case. I think this is the case for most professional icon artists. In fact, many design at much larger sizes -- 2048x2048, for example -- and scale down elements or the whole image as necessary. You're never sure when your icon might have to show up on a billboard or something.
Whether you're making an icon, a poster, or a book cover, you're still just designing graphics. Photoshop and Illustrator are far and away the leaders in 2D graphics design, regardless of the context.
Do you have any recommendations in terms of improving one's design skills?
This is a question I get a lot. As much as I wish I could give a one-line answer, it's tricky because it's so open-ended. It really depends on what your goal is.
One thing you can look at is the video from the UI Design Essentials session we did at CocoaHeads, which was aimed at programmers with that exact question.
Benedikt Terhechte — Sep 02, 08 6324
And, just as you mentioned, the fact that one doesn't need to care about help-files, menu's or interactivity really speeds the development process and aids in concentrating on graphical effects instead. :)
Cheers!
neuro — Sep 05, 08 6340
but again it is too advanced to me , I'm still reading the aaron book (i started reading it two months ago) , and again it is difficult to grasp for beginners people like myself . After looking around , i found the "become an xcoder" it is great introduction but it is not enough to make me ready for Aaron book , then i found your tut in www.cocoadevcentral.com and i loved it for quite some time until you skipped things all the way to the advanced issues i.e quartz and screen savers : ( . I need more basic tutorials please , because i want to be a mac developer. the problem here in riyadh that there is no body that have ever heard about cocoa . but i really want to be a mac developer , did i say it loud enough?! , so how did you become a mac developer yourself? as far as i now that you were a websites designer, is that right?.
If you thing that by reading my post I'm not that kind of person that is gonna make it you must tell me now so i will stop nagging and bin Aaron's book .
thanks
Scott Stevenson — Sep 05, 08 6343
Thanks, though this wasn't really intended as a tutorial. It was mainly a demo of how Core Animation can be helpful for this sort of thing, and I just tossed in a few details about how the effect was done.
i loved it for quite some time until you skipped things all the way to the advanced issues i.e quartz and screen savers
It's a massive task to create a complete set of tutorials that takes somebody from zero desktop programming experience to accomplished Mac programmer. It's complicated by the fact that the tools and frameworks are constantly changing, and need to be updated on a regular basis (which takes time away from writing new material, and vice versa).
The time to create one of the tutorials on Cocoa Dev Central is usally about one-to-three weeks, depending on the complexity of the topic. There are tons of iterations before the finished product is delivered. I spend a lot of time trying to figure out which parts can be cut, which things need to be added, how I can reorganize the thing so it flows more cleanly. Even though the final result may seem pretty simple to read, it's generally a lot of work to get it to that point.
Now I really enjoy doing it, so it's not a matter of desire. The thing is that even with donations, it doesn't come close to providing a reasonable income. So it's not something I can do all the time. So instead of writing a complete series, I try to pick the topics that I think will be most helpful to programmers right now. I also try to provide a mix of beginner and more advanced material.
In other words, it's not just absolute beginners that need help. There are accomplished, veteran programmers that are looking for tutorials on Cocoa as well.
so how did you become a mac developer yourself?
I learned Java first, using Bruce Eckel's Thinking in Java. That book helped cement the object-oriented concepts for me. After that, I read Aaron Hillegass's Cocoa Programming for Mac OS X, then starting working on a Cocoa project. I learned C somewhere in there as well, but I don't remember when exactly.
It certainly helped me to have access to other Cocoa programmers here in Cupertino, but that was before internet-based Cocoa mailing lists, forums, and blogs had really taken off. I think StepWise was the only big one around then.
Mark — Sep 30, 08 6418
Scott Stevenson — Oct 02, 08 6422
I could, yes, but it would probably take a full work day to revamp all of the code and package it into something readable. I hacked a lot of it together since I didn't have much time and it was only going to be used once.
David Vandevoorde — Oct 03, 08 6444
If you're interested in typos, one example has "mosiacConainerLayer" (I assume you meant "mosaicContainerLayer" and another "mosiacTileLayer" (I'm guessing you meant "mosaicTileLayer")
jmndos — Oct 08, 08 6474
Hey, do you have any kiosk example, such as the one on TN2062. Everything is broken on that site and apple wont fix it.
Also, how did you make the background completely black ?
Scott Stevenson — Oct 08, 08 6475
No, I don't have anything like that. Sorry.
Everything is broken on that site and apple wont fix it.
If you file a bug report I'm sure someone will look at it.
Also, how did you make the background completely black ?
You can just set the backgroundColor property of the parent layer to a black CGColorRef.
Roman Busyghin — Oct 12, 08 6482
Scott Stevenson — Oct 13, 08 6484
It looks fine to me, other than the fact that it's not exactly identical to the effect I used. The main difference seems to be that my code moved the layers to the bottom of the screen rather than having them fly towards the viewer. Am I missing your point?
Roman Busyghin — Oct 14, 08 6488